Adding scripts
Introduction
The editor allows to add scripts to your project in order to add interactivity to your scenes. The scripts are written in TypeScript and consist on 2 main methods:
- onStart: Called when the script is loaded and the scene is ready.
- onUpdate: Called each time a frame is rendered on the screen.
Scripts are made to be attached to objects and multiple scripts can be attached to the same object. Linked with the babylonjs-editor-tools package installed with the project, some useful decorators are available to help retrieving objects and customizing the scripts.
This feature is still Work in progress and some features like decorators are not yet available for function-based scripts.
Scripts can be written using both methods class-based:
import { Mesh } from "@babylonjs/core/Meshes/mesh";
export default class MyScriptComponent {
public constructor(public mesh: Mesh) { }
public onStart(): void {
// Do something when the script is loaded
}
public onUpdate(): void {
// Executed each frame
this.mesh.rotation.y += 0.04 * this.mesh.getScene().getAnimationRatio();
}
}
and function-based:
import { Mesh } from "@babylonjs/core/Meshes/mesh";
export function onStart(mesh: Mesh): void {
// Do something when the script is loaded
}
export function onUpdate(mesh: Mesh): void {
// Executed each frame
mesh.rotation.y += 0.04 * mesh.getScene().getAnimationRatio();
}
Adding script
The first steps consists on creating a new script before it can be applied on an object.
To do so, right-click somewhere in the src folder of the project using the Assets Browser panel in the editor and select Add -> Script -> Class based or Add -> Script -> Function based
To do so, right-click somewhere in the src folder of the project using the Assets Browser panel in the editor and select Add -> Script -> Class based or Add -> Script -> Function based
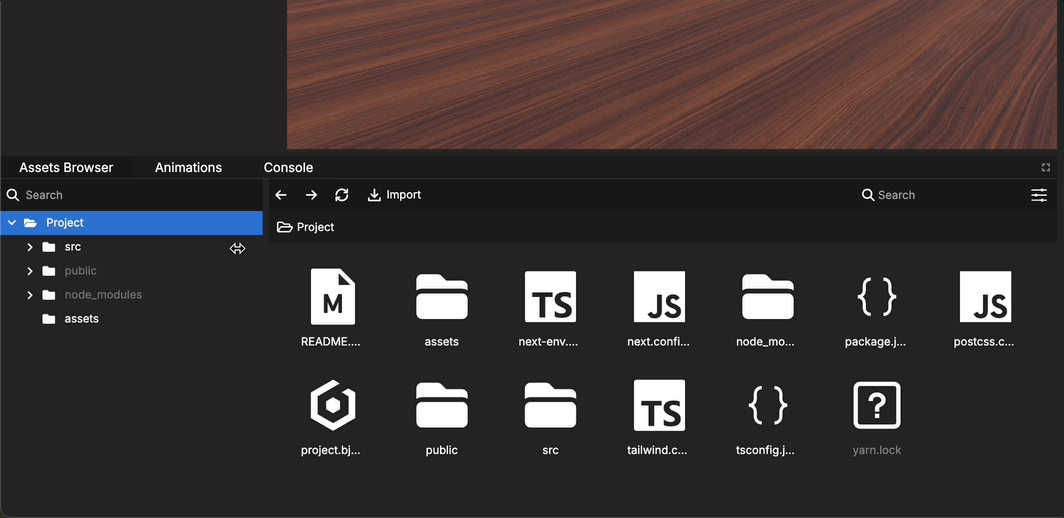
Attaching script
Once a script is available in the sources folder, just select an object in the scene (a mesh for example) so the inspector shows the properties of the mesh and then drag'n'drop the script file from the Assets Browser panel to the Scripts section in the inspector.
Once done, the script is attached to be object and will be executed automatically when running the application.
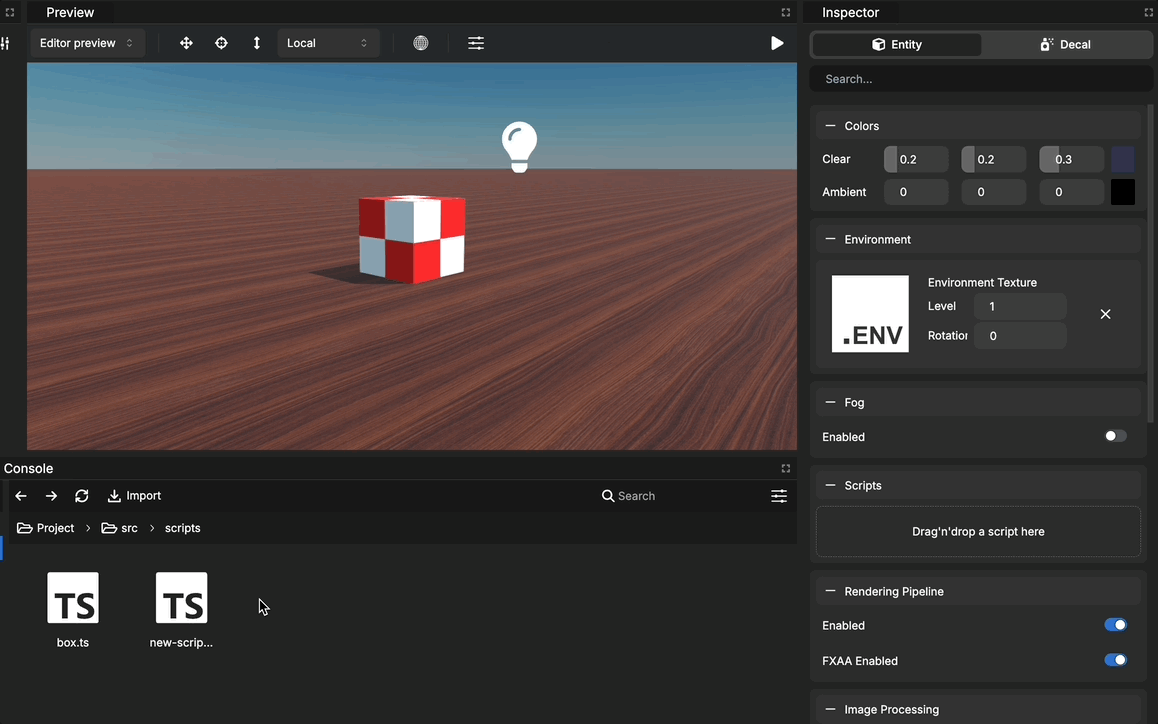
Using decorators
The babylonjs-editor-tools package provides some useful decorators to help retrieving objects and customizing the scripts.
Available decorators are:
- @nodeFromScene: Retrieve the reference of the first node that has the given name by traversing the entire scene graph.
- @nodeFromDescendants: Retrieve the reference of the first node that has the given name but only if the node is a descendant of the object the script is attached to.
- @particleSystemFromScene: Retrieve the reference of the first particle system that has the given name by traversing the entire scene graph.
- @soundFromScene: Retrieve the reference of the first sound that has the given name.
Those decorators are equivalent to calling the associated methods like scene.getMeshById("..."), scene.getTransformNodeById("...").
Example:
import { Mesh } from "@babylonjs/core/Meshes/mesh";
import { Sound } from "@babylonjs/core/Audio/sound";
import { ParticleSystem } from "@babylonjs/core/Particles/particleSystem";
import {
nodeFromScene,
nodeFromDescendants,
particleSystemFromScene,
soundFromScene,
} from "babylonjs-editor-tools";
export default class MyScriptComponent {
@nodeFromScene("ground")
private _ground: Mesh;
@nodeFromDescendants("box")
private _box: Mesh;
@particleSystemFromScene("particles")
private _particleSystem: ParticleSystem;
@soundFromScene("assets/sound.mp3")
private _mySound: Sound;
...
}